In this tutorial, I will give you an example of “Generate Random Unique String in Laravel”, So you can easily apply it with your laravel 5, laravel 6, laravel 7, and laravel 8 application.
Unique String is mostly used to manage Products, Orders, and Invoices for identifying using his random id on bills or invoices in the application.
Related Article : <a href="https://8bityard.com/how-to-generate-unique-order-numbers-in-laravel-quick-ea/" target="_blank" rel="noreferrer noopener">Generate unique Order numbers in Laravel.</a>
First, what we’re doing here, This is the example :
Laravel provides multiple string helpers, We use substr(md5(mt_rand()) helper of Laravel. It is very simple and we can set the limit of string easily.
Syntax:
substr(md5(mt_rand()), 0, 5);
Example:
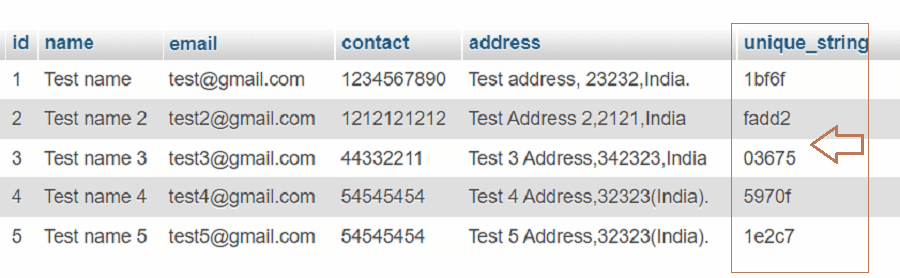
Generating Migration
At first, we create a new migration file for “unique_string_examples” table, In your cmd terminal hit the given below command
php artisan make:migration create_unique_string_examples_table
Migration Structure
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateUniqueStringExamples extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('unique_string_examples', function (Blueprint $table) {
$table->id();
$table->string('name',25);
$table->string('email',25);
$table->string('contact',25);
$table->string('address',50);
$table->string('unique_string',25);
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('unique_string_examples');
}
}
Run Migration
After defining columns and data types For migrating in the cmd terminal hit the given below command.
php artisan migrate
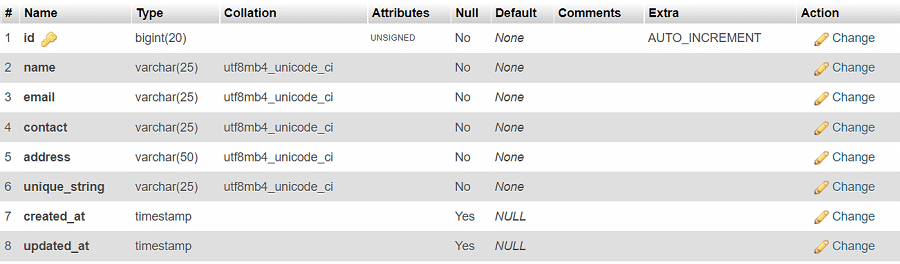
Create a Model
php artisan make:model UniqueStringExample
App\UniqueStringExample.php
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
class UniqueStringExample extends Model
{
protected $table = 'unique_string_examples';
}
Create Controller
php artisan make:controller TestController
routes\web.php
use App\Http\Controllers\TestController;
Route::get('/add', [TestController::class,'add'])->name('add.string');
Route::post('/store',[TestController::class,'store'])->name('store.string');
Http\Controllers\TestController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\UniqueStringExample;
class TestController extends Controller
{
#Generate random string in Laravel
public function add(){
return view('randomstringexample');
}
public function store(Request $request){
$data = new UniqueStringExample();
$data->name = $request->name;
$data->email = $request->email;
$data->contact = $request->contact;
$data->address = $request->address;
$data->unique_string = substr(md5(mt_rand()), 0, 5);
$data->save();
dd('success');
}
}
resources\views\randomstringexample.blade.php
<div class="container">
<div class="row">
<div class="col-md-8 offset-2 mt-5">
<div class="card">
<div class="card-header bg-info">
<h6 class="text-white">How to Generate Unique String | Laravel</h6>
</div>
<div class="card-body">
<form method="post" action="{{route('store.string')}}">
@csrf
<div class="form-group">
<label>Name</label>
<input type="text" name="name" class="form-control"/>
</div>
<div class="form-group">
<label>Email</label>
<input type="email" name="email" class="form-control"/>
</div>
<div class="form-group">
<label>Contact</label>
<input type="text" name="contact" class="form-control"/>
</div>
<div class="form-group">
<label>Address</label>
<input type="text" name="address" class="form-control"/>
</div>
<div class="form-group text-center">
<button type="submit" class="btn btn-info">Submit</button>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
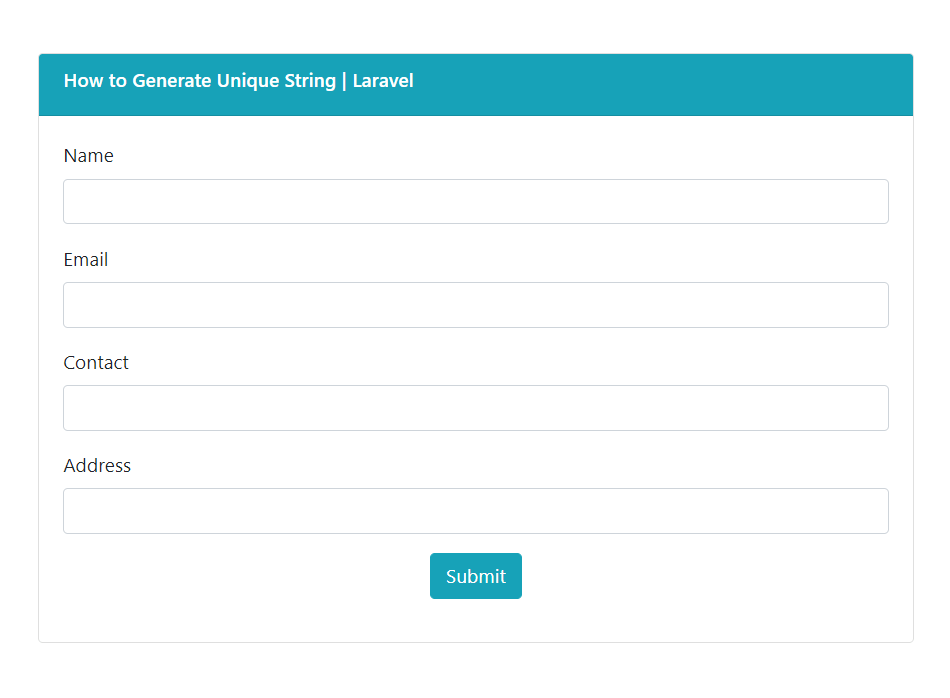
Now, you can see the output in the blade file, we have successfully Generated Random Unique String in Laravel using PHP in the Laravel application.
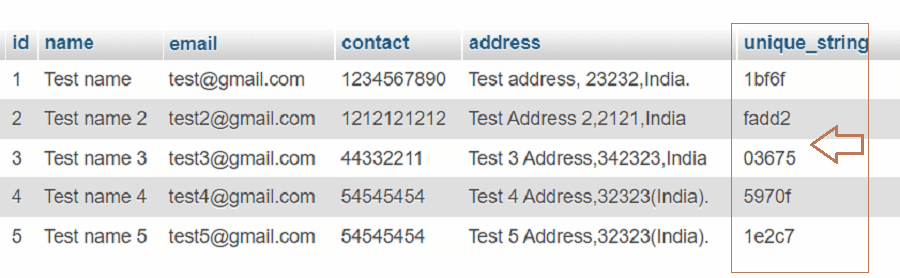
In this article, we learned “How to create a unique random string in Laravel”, I hope this article will help you with your application Project.
Also Read: Limit the length of a string in Blade file Laravel.