Do you want to Display Laravel Array Validation: Set Messages with Position/Index in the Laravel application?
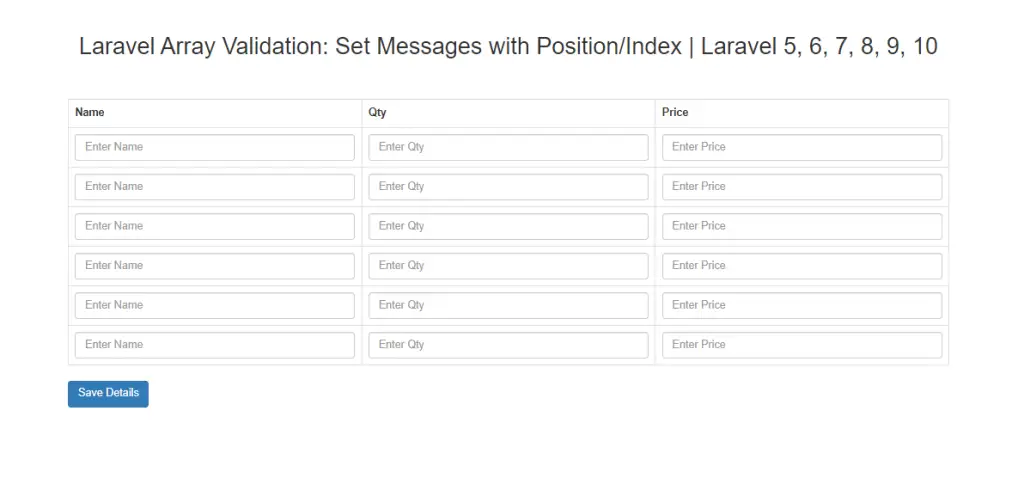
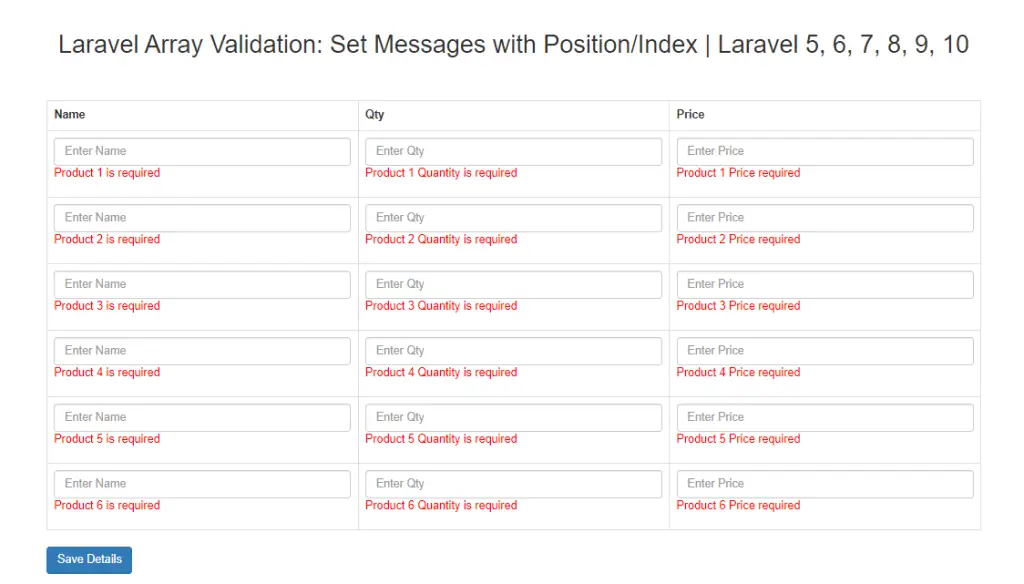
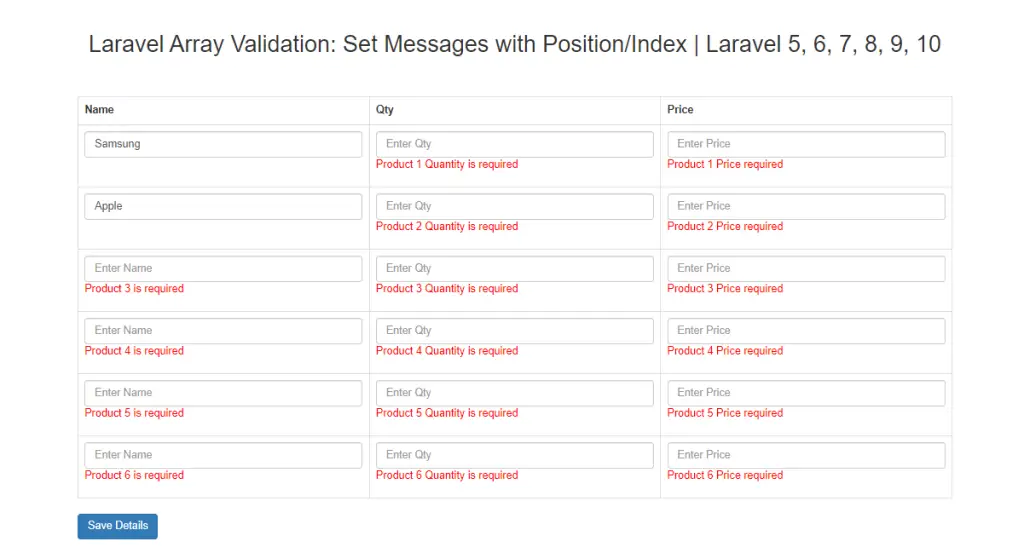
This step-by-step tutorial helps you learn Laravel Array Validation: Set Messages with Position/Index Laravel array validation to get an index in the Laravel application with the help of the Validate array keys in Laravel.
Need of Laravel array validation to get index
The array rule accepts a list of allowed array keys, When validating arrays, we may want to reference the index or position of a particular item that failed validation within the error message displayed by your application. To accomplish this, we may include the : index (starts from 0) and : position (starts from 1).
routes\web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ProductController;
Route::view('/create-product','create');
Route::post('/store-product',[ProductController::class,'storeProduct'])->name('product.store');
resources\views\create.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Laravel Array Validation: Set Messages with Position/Index</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<style>
p.error {
color: red;
}
</style>
</head>
<body>
<br><br>
<div class="container">
<h2 align="center">Laravel Array Validation: Set Messages with Position/Index | Laravel 5, 6, 7, 8, 9, 10</h2>
<br><br>
<form action="{{ route('product.store') }}" method="POST">
@csrf
<table class="table table-bordered" id="dynamicTable">
<tr>
<th>Name</th>
<th>Qty</th>
<th>Price</th>
</tr>
@for($i=0; $i <= 5; $i++)
<tr>
<td>
<input type="text" name="products[{{ $i }}][name]" value="{{ old('products.'.$i.'.name') }}" placeholder="Enter Name" class="form-control" />
@error('products.'.$i.'.name')
<p class="error"> {{ $message }}</p>
@enderror
</td>
<td>
<input type="text" name="products{{ $i }}[qty]" value="{{ old('products.'.$i.'.qty') }}" placeholder="Enter Qty" class="form-control" />
@error('products.'.$i.'.qty')
<p class="error"> {{ $message }}</p>
@enderror
</td>
<td>
<input type="text" name="products{{ $i }}[price]" value="{{ old('products.'.$i.'.price') }}" placeholder="Enter Price" class="form-control" />
@error('products.'.$i.'.price')
<p class="error"> {{ $message }}</p>
@enderror
</td>
</tr>
@endfor
</table>
<button type="submit" class="btn btn-primary">Save Details</button>
</form>
</div>
</body>
</html>
Create a validation Request
To handle, validation let’s create a validation request to show error messages
php artisan make:request StoreProductRequest
app\Http\Request\StoreProductRequest.php
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class StoreProductRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return true;
}
/**
* Get the validation rules that apply to the request.
*
* @return array<string, mixed>
*/
public function rules()
{
return [
'products' => ['required','array'],
'products.*.name' => ['required','string'],
'products.*.qty' => ['required','integer'],
'products.*.price' => ['required','integer'],
];
}
public function messages()
{
return [
'products.*.name.required' => __('Product :position is required'),
'products.*.qty.required' => __('Product :position Quantity is required'),
'products.*.price.required' => __('Product :position Price required'),
'products.*.qty.integer' => __('Quantity has to be a number'),
'products.*.price.integer' => __('Price has to be a number'),
];
}
}
Note: position is used to avoid ‘0’ indexing, like (Product 0 is Required).
app\Http\Controllers\ProductController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Http\Requests\StoreProductRequest;
use App\Models\Product;
class ProductController extends Controller
{
public function storeProduct(StoreProductRequest $request)
{
}
}
I hope that this article helped you learn How to handle Error Message Indexes & Positions In Laravel, with the help of the Laravel validation request and validate array keys Laravel example. You may also want to check out our guide on Display hasMany relationships in a Blade File in Laravel example in the Laravel application.