In this tutorial, we will learn how to open confirm model box before deleting items in laravel 8 application. As we know very well delete operation is very command and basic feature of every web application. if you remove an item, post, product, category, etc then it will remove from your browser. So we should always prefer to confirm before removing items in laravel 5, laravel 6, laravel 7, and laravel 8 app.
Sweetalert alert provides us a very simple and better layout for confirming the box, So let’s see below the full example and you can implement confirmation before deleting a record in your web application. you will get a layout like as below screenshot.
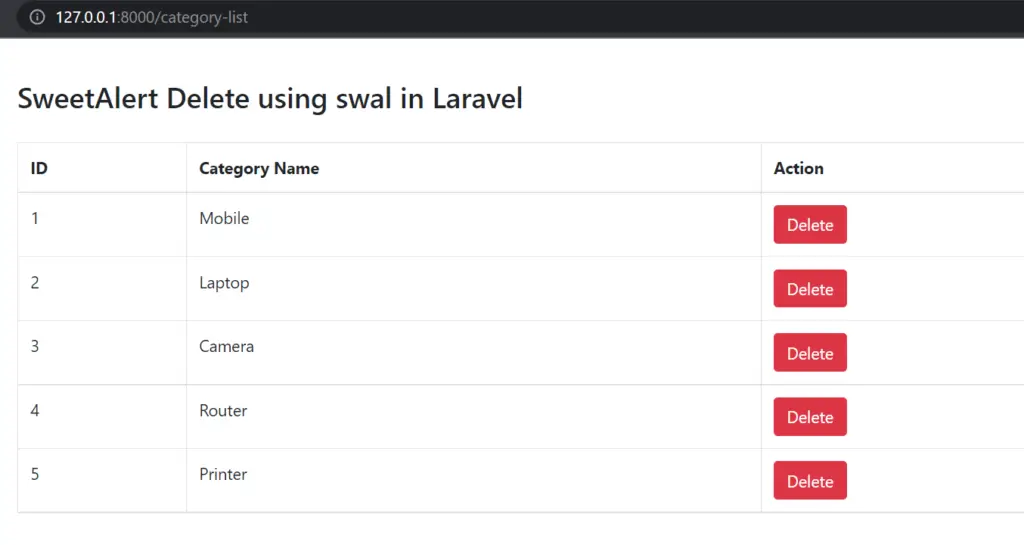
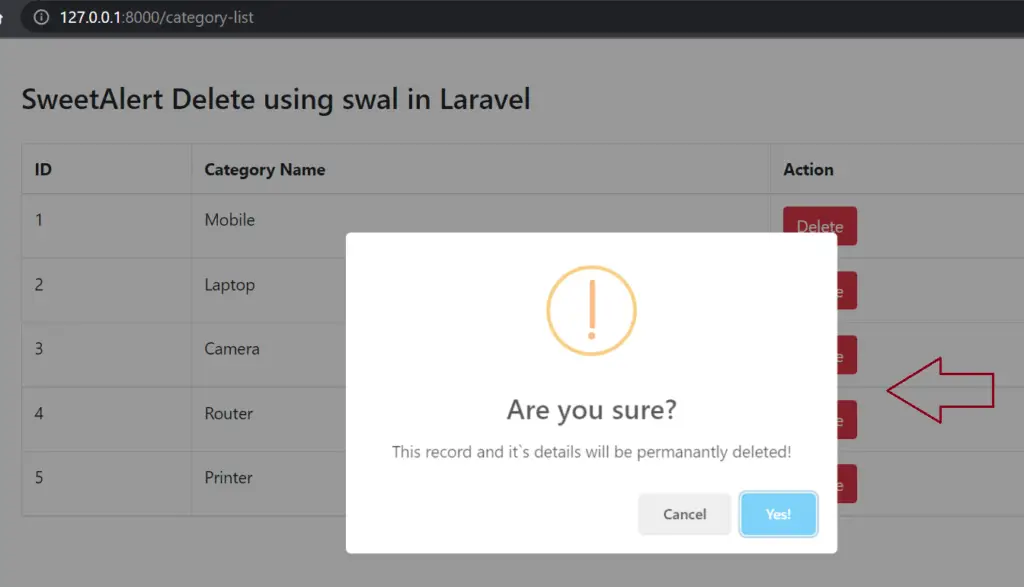
There are several options available to use confirm like jquery UI confirm, sweet alert, boot box, bootstrap confirmation, etc jquery plugin. So we will use swal with sweetalert confirmation before deleting a record.
Installation:
We have a categories table with Category Model, and we have some records in the categories table in the database, we will use the delete method with Sweet alert delete confirm in laravel.
The Best practice is to use sweetalert in the app.blade file and master.blade file using templates, but in this example, We will use sweetalert in the list blade file directly.
To get started with SweetAlert2, use Composer to add this package to laravel project’s dependencies:
composer require realrashid/sweet-alert
Publish the config file :
php artisan sweetalert:publish
Create Controller :
php artisan make:controller TestSweetalertController
routes\web.php :
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\TestSweetalertController;
Route::get('/category-list', [TestSweetalertController::class, 'index']);
Route::get('/category-delete/{id}', [TestSweetalertController::class, 'delete']);
Http\Controllers\TestSweetalertController.php :
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Category;
use RealRashid\SweetAlert\Facades\Alert;
class TestSweetalertController extends Controller
{
public function index()
{
$category = Category::all();
return view('category.list',compact('category'));
}
public function delete($id)
{
$delete = Category::where('id', $id)->delete();
return redirect()->back();
}
}
resources\views\category\list.blade.php :
<!doctype html>
<html lang="en">
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css">
<!-- jquery -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.4.0/jquery.min.js"></script>
<!-- SweetAlert2 -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/sweetalert/2.1.2/sweetalert.min.js"></script>
</head>
<body>
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h3>SweetAlert Delete using swal in Laravel</h3>
</div>
</div>
</div>
<table class="table table-bordered">
<tr>
<th>ID</th>
<th>Category Name</th>
<th>Action</th>
</tr>
@if(isset($category) && !empty($category))
@foreach ($category as $key => $data )
<tr>
<td>{{$key+1}}</td>
<td>{{ $data->name }}</td>
<td>
<a href="/category-delete/{{$data->id}}" class="btn btn-danger delete-confirm" role="button">Delete</a>
</td>
</tr>
@endforeach
@endif
</table>
</body>
</html>
<script>
$('.delete-confirm').on('click', function (event) {
event.preventDefault();
const url = $(this).attr('href');
swal({
title: 'Are you sure?',
text: 'This record and it`s details will be permanantly deleted!',
icon: 'warning',
buttons: ["Cancel", "Yes!"],
}).then(function(value) {
if (value) {
window.location.href = url;
}
});
});
</script>
Add the given below cdn Into your master blade or any blade file where you want to show the delete confirmation message in laravel.
<script src="https://cdnjs.cloudflare.com/ajax/libs/sweetalert/2.1.2/sweetalert.min.js"></script>
You can use the response message or session alerts while you delete your records in your web application.
If you want to add multiple sweetalert attributes in your laravel application, you can check out the sweetalert complete documentation.
In this article, we learned “How to use Sweet alert delete confirm in Laravel 8”, I hope this article will help you with your Laravel application Project.
Read Also: How to Create a Custom 404 error page in Laravel.