To generate and download pdf using dom pdf in Livewire 3 in Laravel, you’ll need to follow a few steps. Dompdf is a popular PHP library that converts HTML and CSS to PDF. Here’s a step-by-step guide:
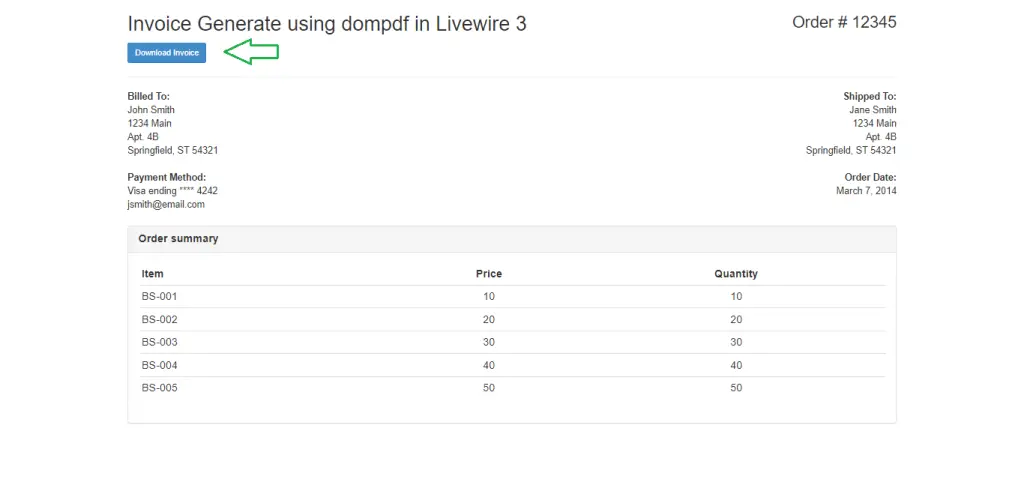
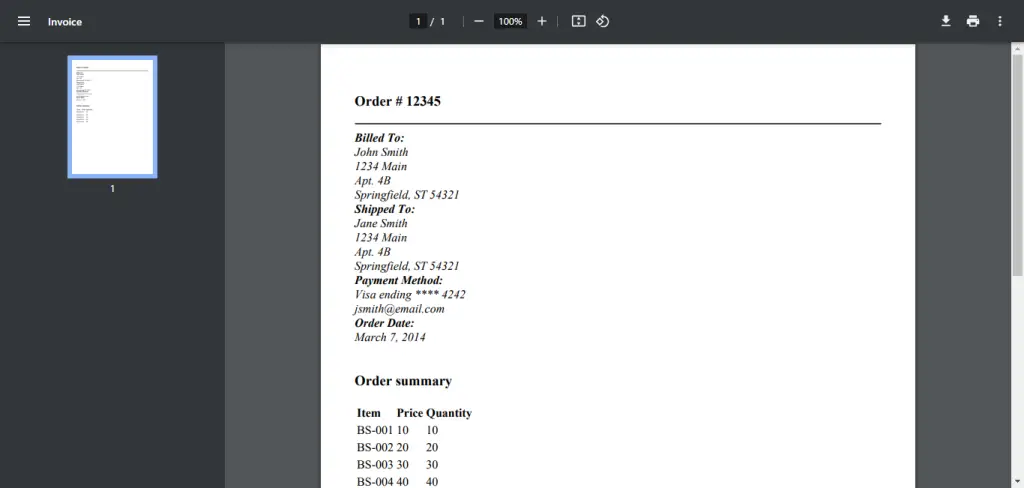
Install Dompdf via Composer:
Open your terminal and navigate to your Livewire Laravel project directory. Run the following command to install Dompdf:
composer require barryvdh/laravel-dompdf
Publish the configuration file:
Run the following command to publish the configuration file:
php artisan vendor:publish --provider="Barryvdh\DomPDF\ServiceProvider"
Create a Livewire Component:
Create a new livewire component using the following command:
php artisan make:livewire PdfInvoice
Setup the layout file:
resources\layouts\app.blade.php
<html>
<head>
@livewireStyles
@stack('css')
</head>
<body>
@yield('content')
@livewireScripts
@stack('script')
</body>
</html>
Edit the PdfInvoice.php
file to include a method for generating and downloading the PDF. For example:
Livewire\PdfInvoice.php
<?php
namespace App\Livewire;
use Barryvdh\DomPDF\Facade\Pdf;
use Livewire\Component;
class PdfInvoice extends Component
{
public $order_details = [
['item_code' => 'BS-001', 'price' => '10','quantity' => '10'],
['item_code' => 'BS-002', 'price' => '20','quantity' => '20'],
['item_code' => 'BS-003', 'price' => '30','quantity' => '30'],
['item_code' => 'BS-004', 'price' => '40','quantity' => '40'],
['item_code' => 'BS-005', 'price' => '50','quantity' => '50'],
];
public function downloadInvoice()
{
$pdf = Pdf::loadView('invoice_pdf',
['invoice_data' => $this->order_details ?? '']);
$inv_no = random_int(1000, 9999);
return response()->streamDownload(function () use($pdf) {
echo $pdf->stream();
}, $inv_no."_".'invoice.pdf');
}
public function render()
{
return view('livewire.pdf-invoice')->extends('layouts.app');
}
}
routes\web.php
<?php
use Illuminate\Support\Facades\Route;
use App\Livewire\PdfInvoice;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider and all of them will
| be assigned to the "web" middleware group. Make something great!
|
*/
Route::get('/invoice', PdfInvoice::class)->name('pdf.invoice');
livewire\pdf-invoice.blade.php
<div>
@push('css')
<link href="//netdna.bootstrapcdn.com/bootstrap/3.1.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css">
<style>
.invoice-title h2, .invoice-title h3 {
display: inline-block;
}
.table > tbody > tr > .no-line {
border-top: none;
}
.table > thead > tr > .no-line {
border-bottom: none;
}
.table > tbody > tr > .thick-line {
border-top: 2px solid;
}
</style>
@endpush
<div class="container">
<div class="row">
<div class="col-xs-12">
<div class="invoice-title">
<h2>Invoice Generate using dompdf in Livewire 3</h2>
<h3 class="pull-right">Order # 12345</h3>
</div>
<div>
<button type="button" wire:click="downloadInvoice" class="btn btn-primary btn-sm">
Download Invoice
</button>
</div>
<hr>
<div class="row">
<div class="col-xs-6">
<address>
<strong>Billed To:</strong><br>
John Smith<br>
1234 Main<br>
Apt. 4B<br>
Springfield, ST 54321
</address>
</div>
<div class="col-xs-6 text-right">
<address>
<strong>Shipped To:</strong><br>
Jane Smith<br>
1234 Main<br>
Apt. 4B<br>
Springfield, ST 54321
</address>
</div>
</div>
<div class="row">
<div class="col-xs-6">
<address>
<strong>Payment Method:</strong><br>
Visa ending **** 4242<br>
jsmith@email.com
</address>
</div>
<div class="col-xs-6 text-right">
<address>
<strong>Order Date:</strong><br>
March 7, 2014<br><br>
</address>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-md-12">
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title"><strong>Order summary</strong></h3>
</div>
<div class="panel-body">
<div class="table-responsive">
<table class="table table-condensed">
<thead>
<tr>
<td><strong>Item</strong></td>
<td class="text-center"><strong>Price</strong></td>
<td class="text-center"><strong>Quantity</strong></td>
</tr>
</thead>
<tbody>
@foreach($order_details as $item)
<tr>
<td>{{ $item['item_code'] }}</td>
<td class="text-center">{{ $item['price'] }}</td>
<td class="text-center">{{ $item['quantity'] }}</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
@push('script')
<script src="//netdna.bootstrapcdn.com/bootstrap/3.1.0/js/bootstrap.min.js"></script>
<script src="//code.jquery.com/jquery-1.11.1.min.js"></script>
@endpush
</div>
Create a Blade View for Pdf:
resources/views/invoice_pdf.blade.php In this file, you can write the HTML and CSS content that you want to convert to PDF.
<!DOCTYPE html>
<html>
<head>
<title>Invoice</title>
</head>
<body>
<div class="row">
<div class="col-xs-12">
<div class="invoice-title">
<h3 class="pull-right">Order # 12345</h3>
</div>
<hr>
<div class="row">
<div class="col-xs-6">
<address>
<strong>Billed To:</strong><br>
John Smith<br>
1234 Main<br>
Apt. 4B<br>
Springfield, ST 54321
</address>
</div>
<div class="col-xs-6 text-right">
<address>
<strong>Shipped To:</strong><br>
Jane Smith<br>
1234 Main<br>
Apt. 4B<br>
Springfield, ST 54321
</address>
</div>
</div>
<div class="row">
<div class="col-xs-6">
<address>
<strong>Payment Method:</strong><br>
Visa ending **** 4242<br>
jsmith@email.com
</address>
</div>
<div class="col-xs-6 text-right">
<address>
<strong>Order Date:</strong><br>
March 7, 2014<br><br>
</address>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-md-12">
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title"><strong>Order summary</strong></h3>
</div>
<div class="panel-body">
<div class="table-responsive">
<table class="table table-condensed">
<thead>
<tr>
<td><strong>Item</strong></td>
<td class="text-center"><strong>Price</strong></td>
<td class="text-center"><strong>Quantity</strong></td>
</tr>
</thead>
<tbody>
@foreach($invoice_data as $item)
<tr>
<td>{{ $item['item_code'] }}</td>
<td class="text-center">{{ $item['price'] }}</td>
<td class="text-center">{{ $item['quantity'] }}</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
Run application:
http://127.0.0.1:8000/invoice
Open your browser and navigate to http://127.0.0.1:8000/invoice This should trigger the downloadInvoice method, generate the PDF, and prompt you to download it.
Remember to customize the views, data, and routes according to your application’s requirements. This is a basic example to get you started with Generate and download pdf using dom pdf in Livewire 3 in the Laravel application.
Read also: How to add custom font in dompdf in Laravel.