Today, I will give you an example of “How to Make Delete Method API in Laravel”, So you can easily apply it with your laravel 5, laravel 6, laravel 7, laravel 8, and Laravel 9 application.
First, what we’re doing here, This is the example :
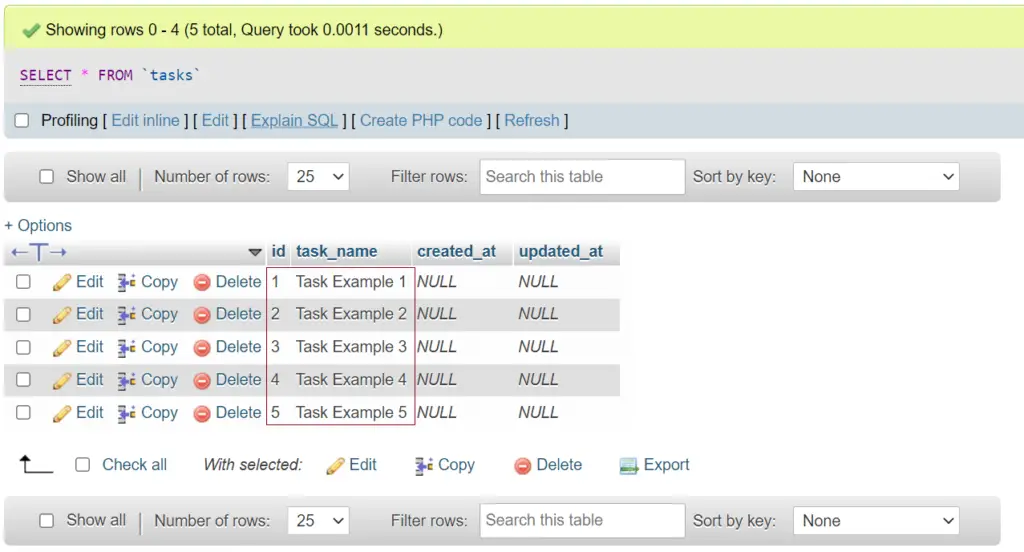
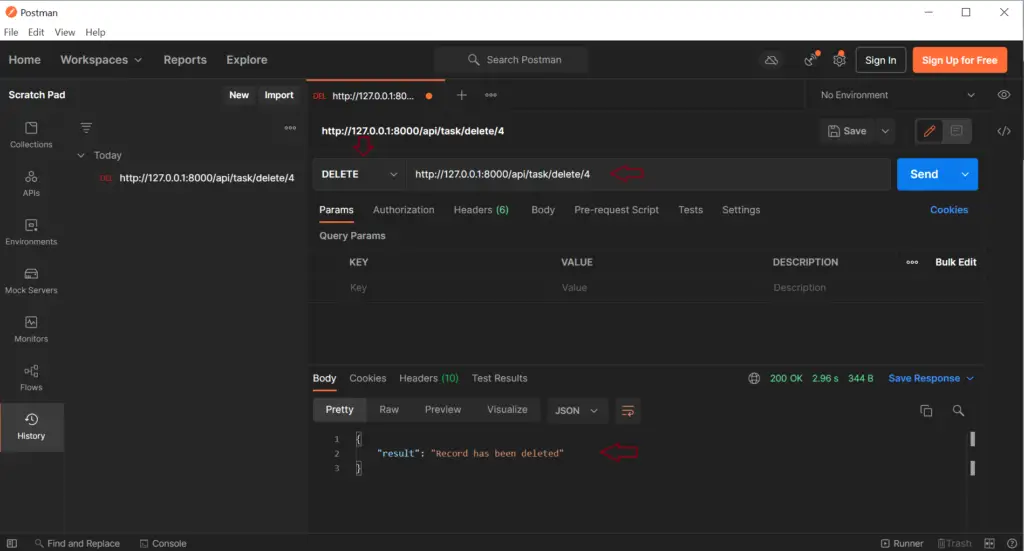
Need of Delete Method API in Laravel
We use delete API to delete records in our table in Laravel. In this example, We will pass a parameter id from the API route URL, and we will get the id in our controller using a function, and using that ID we will delete the related ID records from our table using the Postman API Platform.
Let’s get started.
Generating Migration with Model
php artisan make:model Task -m
Migration Structures
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateTasksTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('tasks', function (Blueprint $table) {
$table->id();
$table->string('task_name');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('tasks');
}
}
Run Migration
php artisan migrate
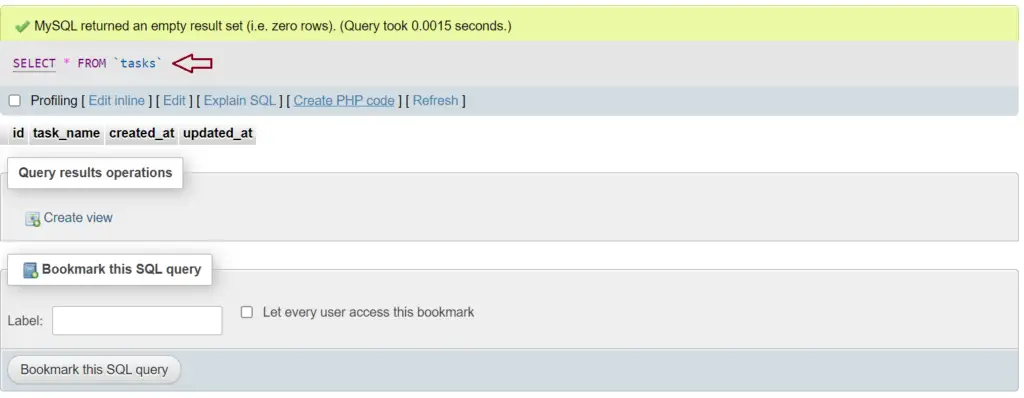
app\Models\Task.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Task extends Model
{
use HasFactory;
}
Create a Controller
php artisan make:controller TaskController
Create a database Seeder
We create a Database Seeder to Insert some Dummy records in our tasks table.
php artisan make:seeder TaskTableSeeder
database\seeders\TaskTableSeeder.php
<?php
namespace Database\Seeders;
use Illuminate\Database\Seeder;
use App\Models\Task;
class TaskTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
$tasks =[
['id'=>1,'task_name'=>'Task Example 1'],
['id'=>2,'task_name'=>'Task Example 2'],
['id'=>3,'task_name'=>'Task Example 3'],
['id'=>4,'task_name'=>'Task Example 4'],
['id'=>5,'task_name'=>'Task Example 5']
];
Task::insert($tasks);
}
}
Register Seeder
We Register TaskTableSeeder Class in DatabaeSeeder File.
database\seeders\DatabaseSeeder.php
<?php
namespace Database\Seeders;
use Illuminate\Database\Seeder;
class DatabaseSeeder extends Seeder
{
/**
* Seed the application's database.
*
* @return void
*/
public function run()
{
// \App\Models\User::factory(10)->create();
$this->call(TaskTableSeeder::class);
}
}
Hit the Seed Command
php artisan db:seed
Define API Routes
routes/api.php
<?php
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\TaskController;
/*
|--------------------------------------------------------------------------
| API Routes
|--------------------------------------------------------------------------
|
| Here is where you can register API routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| is assigned the "api" middleware group. Enjoy building your API!
|
*/
Route::middleware('auth:api')->get('/user', function (Request $request) {
return $request->user();
});
Route::delete('/task/delete/{id}',[TaskController::class, 'delete']);
app\Http\Controllers\TaskController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Task;
class TaskController extends Controller
{
public function delete($id)
{
$task = Task::findOrFail($id);
$result = $task->delete();
if($result){
return ['result'=>'Record has been deleted'];
}
}
}
Setup and Test Postman for Delete API
We test our delete API in Postman, please copy your API URL Select the Delete option in postman and pass an ID, and hit the send button.
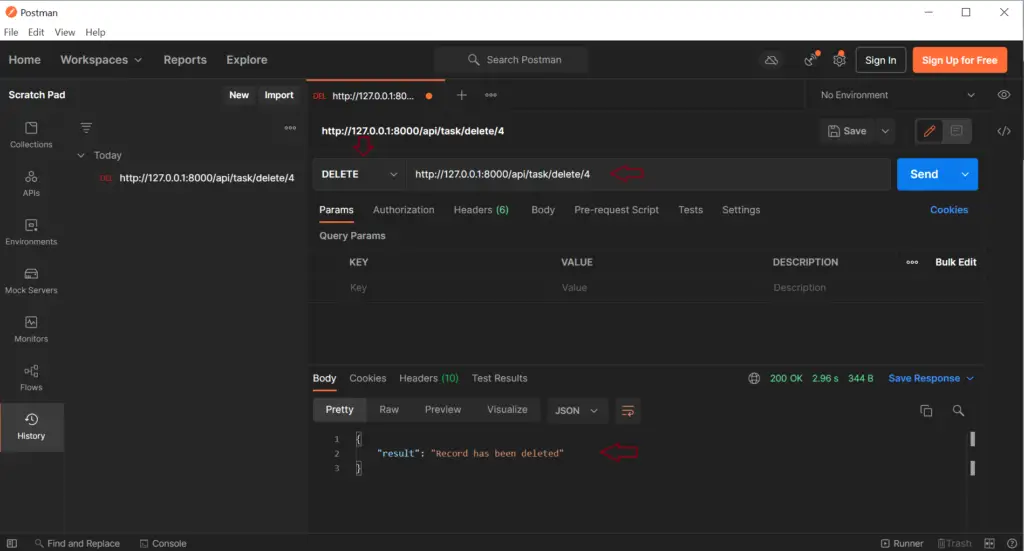
I am passing the ID 4, you can see when I send the request, the ID 4 related record is deleted from my tasks table successfully with a success message.
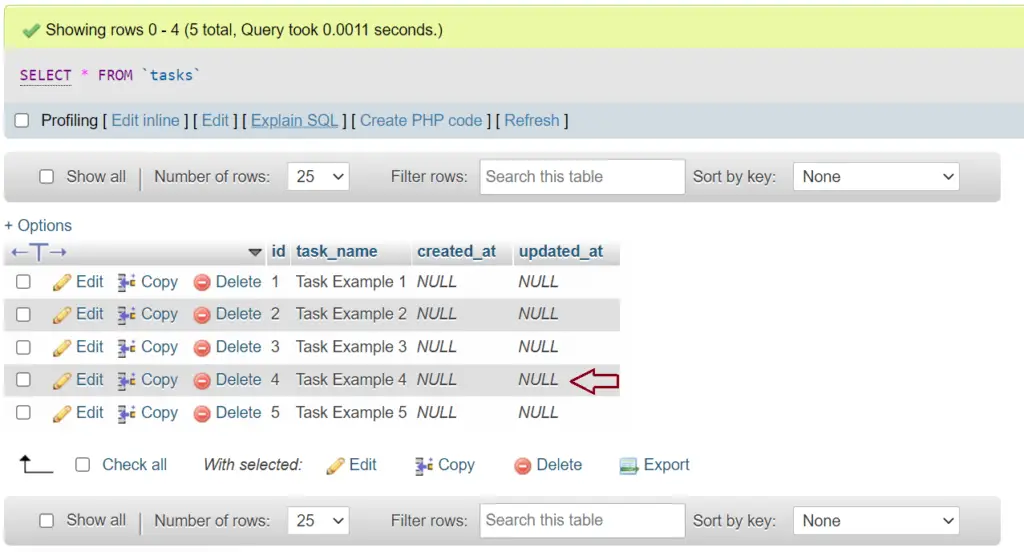
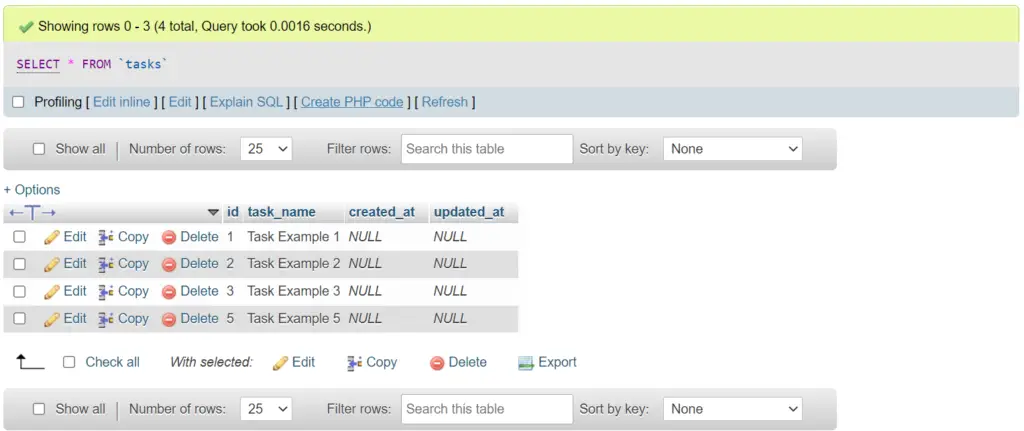
In case the ID record is not found in our table it will throw us to the error page, and you can also handle this using the try-catch exception or pass an error message in your controller.
Related Post: Validate API in Laravel.
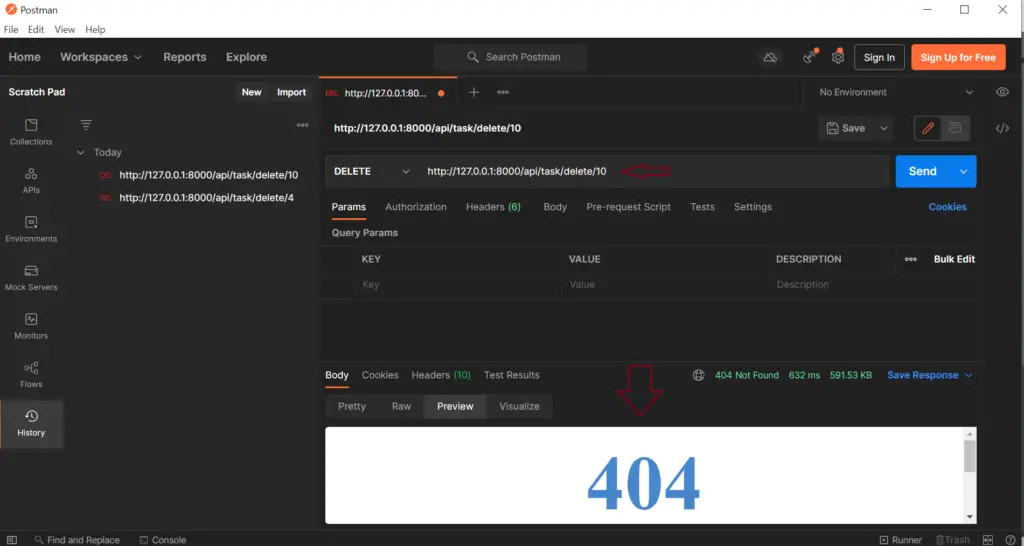
In this article, we successfully learned “How to make Delete API in Laravel”, I hope this article will help you with your Laravel application Project.
Also Read: Get the Title and Description of any URL in Laravel.